var fs = require('fs')
// create a random file with random data
var write = fs.writeFileSync('input.txt', "Written to input.txt")
// create read stream *******************************
var stream = fs.createReadStream("input.txt");
// set encoding
stream.setEncoding('utf8')
// handle the events of read stream i.e. data , end , error
var data = '';
// data event
stream.on('data', function(chunk) {
data += chunk;
})
// end event
stream.on('end', function() {
console.log(data);
})
// error event
stream.on('error', function(err) {
console.log(err);
})
// create writeStream ***************************
var write = fs.createWriteStream('output.txt');
// create a variable with random data
var data = 'data to be written to output.txt file'
// write data to output file with utf8 encoding
write.write(data, 'utf8')
// handle events of the write stream finish, error
// finish
write.on('finish', function() {
console.log("Data Written");
})
// error event
write.on('error', function(err) {
console.log("Error Writing data")
})
// using pipe ***************** Used to read from one stream and write to another stream
// create a read stream
var readPi = fs.createReadStream('input.txt');
var writePi = fs.createWriteStream('outputnew.txt');
readPi.pipe(writePi);
// handle events of readPi and writePi
readPi.on("error", function(error) {
console.log(error.stack)
})
readPi.on("end", function() {
console.log("Reading Completed for piping")
})
writePi.on("finish", function() {
console.log("Writing completed for piping")
})
writePi.on("error", function() {
console.log(error.stack)
})
Node.js Stream Example
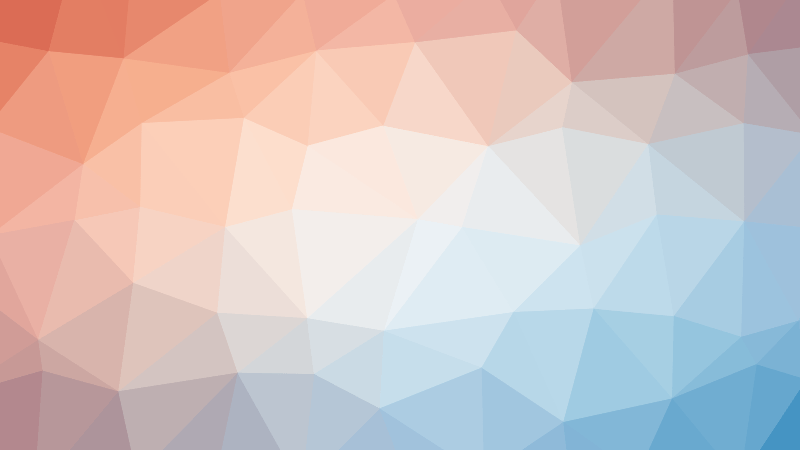