#include < stdio.h >
#include < stdlib.h >
int main() {
printf("Enter the number of elements\n");
int total;
scanf("%d", & total);
int arr[total];
for (int temp = 0; temp < total; temp++) {
printf("\n Enter the number %d: ", temp + 1);
scanf("%d", & arr[temp]);
}
// bubble sort
for (int temp = 0; temp < total; temp++) {
for (int tempp = temp + 1; tempp < total; tempp++) {
if (arr[temp] > arr[tempp]) {
//swap
int tempVar = arr[temp];
arr[temp] = arr[tempp];
arr[tempp] = tempVar;
}
}
}
printf("\n");
// print array
for (int temp = 0; temp < total; temp++) {
printf("%d,", arr[temp]);
}
return 0;
}#include < stdio.h > #include < stdlib.h >
struct node {
int value;
struct node * left;
struct node * right;
};
void add(struct node * root, int val);
struct node * create(int s);
void traverse(struct node * root);
void print_depth(struct node * root, int * TotalDepth, int currentDepth);
void main() {
int total, num, i;
struct node * root;
printf("hello");
printf("Enter the number of values in tree\n");
scanf("%d", & total);
if (total <= 0) return;
// enter values in tree
for (i = 0; i < total; i++) {
printf("Enter value No. %d\n", i + 1);
scanf("%d", & num);
if (i == 0) root = create(num);
else {
add(root, num);
}
}
// traverse
traverse(root);
printf("\n");
int depth = 0, currentDepth = 0;
print_depth(root, & depth, currentDepth);
// we have to minus 1 from depth because root is counted as 0 while in height it counted as 1
printf("The depth is : %d\n", depth - 1);
}
void traverse(struct node * root) {
if (root == NULL) return;
printf("%d", root - > value);
traverse(root - > left);
traverse(root - > right);
}
void add(struct node * root, int val) {
// go to leaf and save
// first check left
if ((root - > value) >= val) {
if ((root - > left) == NULL) {
root - > left = create(val);
return;
} else
add(root - > left, val);
}
// then check right
else if ((root - > value) < val) {
if ((root - > right) == NULL) {
root - > right = create(val);
return;
} else
add(root - > right, val);
}
}
struct node * create(int val) {
struct node * x = (struct node * ) malloc(sizeof(struct node));
x - > value = val;
x - > left = NULL;
x - > right = NULL;
return x;
}
void print_depth(struct node * root, int * TotalDepth, int currentDepth) {
if (root == NULL) return;
if (( * TotalDepth) < (++currentDepth)) {
(++ * TotalDepth);
}
printf("\nTotal:%d,Current:%d", * TotalDepth, currentDepth);
print_depth(root - > left, TotalDepth, currentDepth);
print_depth(root - > right, TotalDepth, currentDepth);
}
Binary Tree Traversal and Depth Find
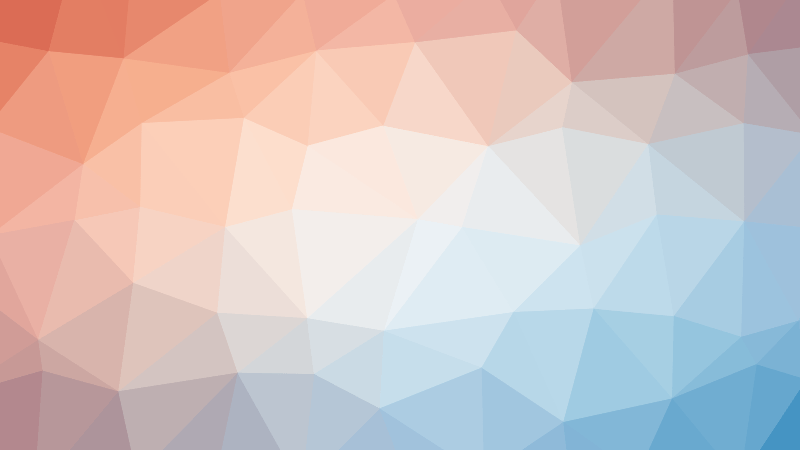