#include < stdio.h >
#include < stdlib.h >
#define ArrayMaxSize 20
void push(int array[], int * currentSize, int * val);
void pop(int * currentSize);
int isEmpty(int * currentSize);
int isFull(int * currentSize, int * userSize);
void showStatus(int array[], int * currentSize, int * userSize);
void printElements(int array[], int * currentSize);
int main() {
int size[ArrayMaxSize], currentSize = 0, userSize;
char choice = 'y';
int check = 1, option;
while (check) {
printf("Enter the Size of the stack.\n Supported Size is 20\n\n");
scanf("%d", & userSize);
if (userSize > 20) {
printf("\nInvalid Size. Press any key to try again");
getch();
} else check = 0;
}
// give choices to do stuff
while (choice == 'y' || choice == 'Y') {
printf("\n\nChoose Option:\n\n");
printf("1. Enter(Push) Element\n");
printf("2. Remove(Pop) Element\n");
printf("3. Check Status\n");
scanf("%d", & option);
if (option == 1) {
printf("\nc = %d, u= %d\n", currentSize, userSize);
// first check if array is full
if (isFull( & currentSize, & userSize) == 1) {
printf("\nThe Stack is Full\n");
} else {
//call the function to push after taking value
int element;
printf("\n\nEnter the number\n");
scanf("%d", & element);
push(size, & currentSize, & element);
}
}
if (option == 2) {
//call the function to remove after taking value
printf("\n\nRemoving the number\n");
pop( & currentSize);
}
if (option == 3) {
//call the function to show status of stack
showStatus(size, & currentSize, & userSize);
}
if (option < 1 || option > 3) {
printf("\nNot a valid option\n");
printf("Press any key to choose again");
getch();
continue;
}
printf("\nDo you want to do more operations\nPress y to confirm\n");
fflush(stdin);
scanf("%c", & choice);
}
printf("\n Thankyou for using this simplest program. Hope to see you again ;-\)\n");
return 0;
}
int isEmpty(int * currentSize) {
if ( * currentSize == 0) return 1;
else return 0;
}
int isFull(int * currentSize, int * userSize) {
if ( * currentSize == * userSize) return 1;
else return 0;
}
void push(int array[], int * currentSize, int * val) {
//push the element
array[ * currentSize] = * val;
++ * currentSize;
printf("\nSuccessfully entered value : %d\n", * val);
}
void pop(int * currentSize) {
// first check for empty condition
if (!isEmpty(currentSize)) {
//pop the element
-- * currentSize;
printf("\nSuccessfully deleted end value");
} else {
printf("\nThe Stack is Empty.\n");
}
}
// function to show current stack status
void showStatus(int array[], int * currentSize, int * userSize) {
if (isFull(currentSize, userSize)) {
printf("\n The Stack is Full and Elements are \n");
printElements(array, currentSize);
return;
}
if (isEmpty(currentSize)) {
printf("The Stack is Empty");
return;
}
// print elements
printElements(array, currentSize);
}
// function to print elements
void printElements(int array[], int * currentSize) {
int i;
printf("\n");
for (i = 0; i < * currentSize; i++) {
printf("%d,", array[i]);
}
printf("\n");
}
Stack implementation in C
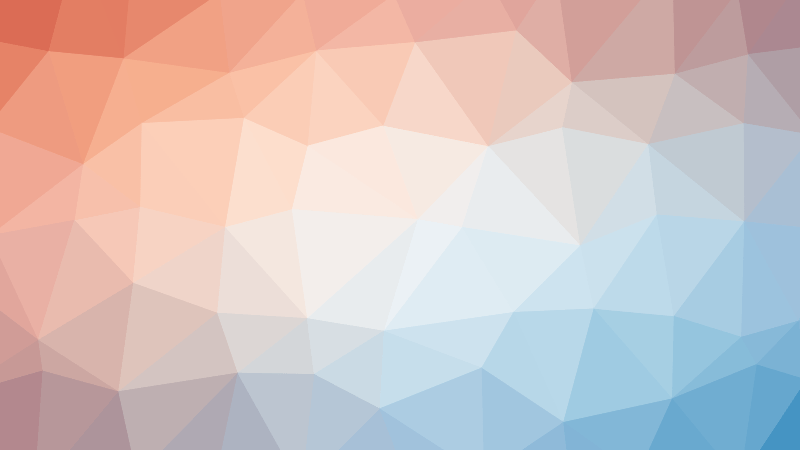