#include < stdio.h >
#include < stdlib.h >
int main() {
printf("Enter the number of elements\n");
int total;
scanf("%d", & total);
int arr[total];
for (int temp = 0; temp < total; temp++) {
printf("\n Enter the number %d: ", temp + 1);
scanf("%d", & arr[temp]);
}
// bubble sort
for (int temp = 0; temp < total; temp++) {
for (int tempp = temp + 1; tempp < total; tempp++) {
if (arr[temp] > arr[tempp]) {
//swap
int tempVar = arr[temp];
arr[temp] = arr[tempp];
arr[tempp] = tempVar;
}
}
}
printf("\n");
// print array
for (int temp = 0; temp < total; temp++) {
printf("%d,", arr[temp]);
}
return 0;
}
Bubble Sort in C
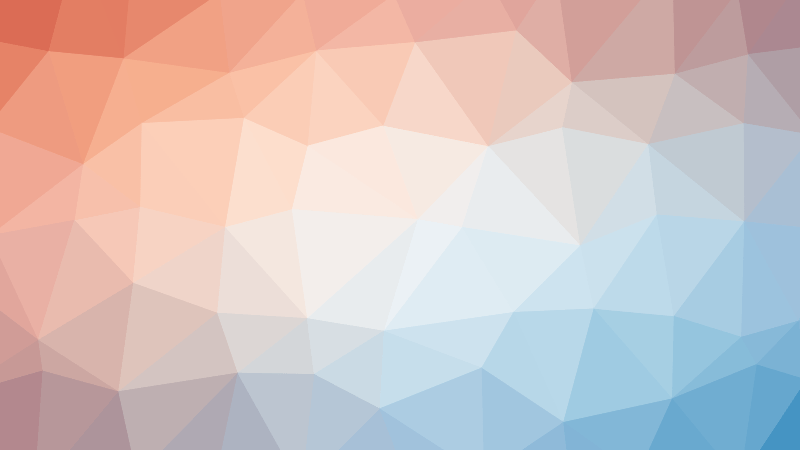