#include < stdio.h >
#include < stdlib.h >
void quickSort(int arr[], int l, int r);
int sortPartition(int arr[], int l, int r);
int main() {
printf("Enter the total Elements");
int total;
scanf("%d", & total);
int arr[total];
for (int i = 0; i < total; i++) {
printf("\nEnter the number %d : ", i + 1);
scanf("%d", arr + i);
}
// call the quick sort
quickSort(arr, 0, total);
for (int i = 0; i < total; i++) {
printf("%d,", arr[i]);
}
return 0;
}
void quickSort(int arr[], int l, int r) {
if (l >= r) return;
int m = sortPartition(arr, l, r);
quickSort(arr, l, m - 1);
quickSort(arr, m + 1, r);
}
int sortPartition(int arr[], int l, int r) {
// take r as pivot and move all the big elements to right and small to left
int min = l - 1;
for (int i = l; i < r; i++) {
if (arr[i] <= arr[r]) {
min++;
// swap i and min
int tempVar = arr[i];
arr[i] = arr[min];
arr[min] = tempVar;
}
}
// and finally swap the pivot r
int tempVar = arr[r];
arr[r] = arr[min + 1];
arr[min + 1] = tempVar;
return min++;
}
Quick Sort in C
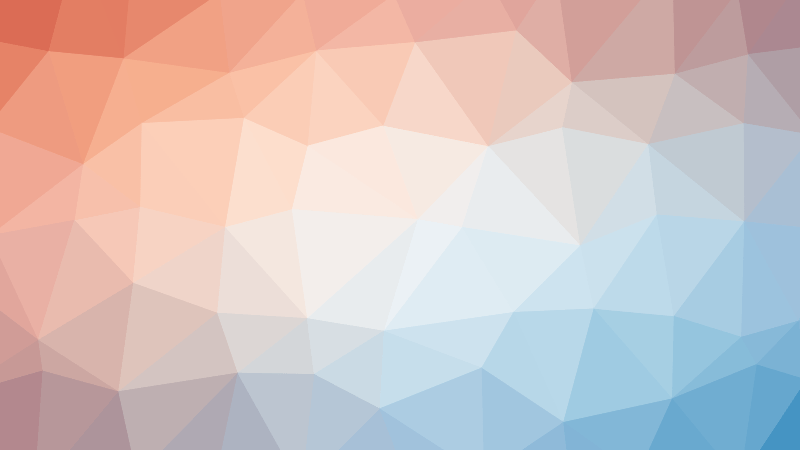