#include < stdio.h >
#include < stdlib.h >
#include < string.h >
int main() {
char * str = "Hi how are you?";
int start = 0;
int end = -1;
int i = 0;
while (i <= strlen(str)) {
if ( * (str + i) == ' ' || * (str + i) == '\0') {
// print from end to start if end>=start
if (end >= start) {
for (int j = end; j >= start; j--) printf("%c", *(str + j));
// make start = end+1 ... now start is pointing to space or \0
start = end + 1;
// print all spaces
while ( * (str + i) == ' ') {
printf(" ");
start++;
i++;
}
// make end = start-1 because end have to be less than start
end = start - 1;
}
} else {
// increment end
end++;
i++;
}
}
return 0;
}
Reverse Words in Given Sentence without using any library method.
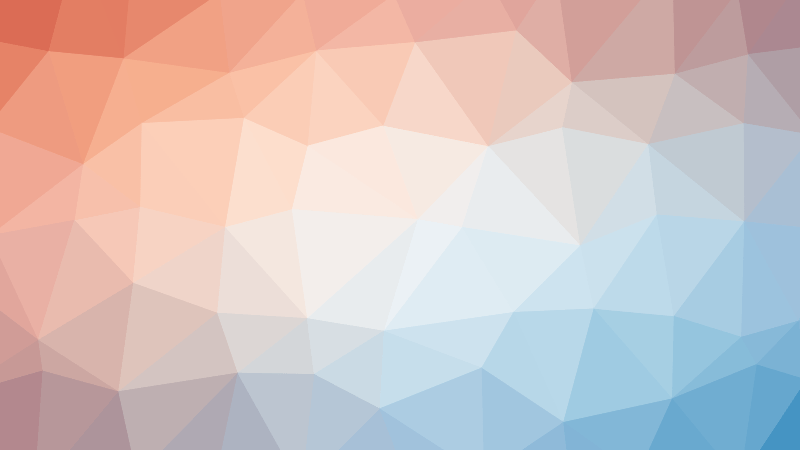