#include < stdio.h >
#include < stdlib.h >
#define print(x) printf("%d", x);
int main() {
int arr[] = {1,2,3,4,5,6,3,4,5,8,5,4,7,5,1,4,5,2,4,5};
// create a auto balancing tree from given numbers for faster execution
// we will use naive method for traversing
// find maximum
int max = 0;
for (int i = 0; i < sizeof(arr) / sizeof(int); i++) {
if ( * (arr + i) > max) max = * (arr + i);
}
int * arrC = malloc(max * sizeof(int));
// make all zero
for (int i = 0; i < max; i++) {
*(arr + i) = 0;
}
// traverse tree... If more than one than do not print
for (int i = 0; i < sizeof(arr) / sizeof(int); i++) {
if ( * (arr + i) == 0) {
printf("%d,", i + 1);*(arr + i) += 1;
}
}
return 0;
}
Find duplicate numbers in array
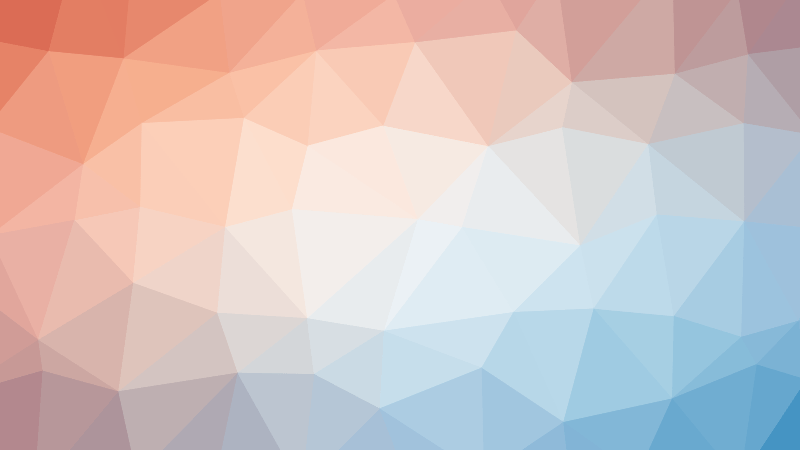