#include < stdio.h >
#include < stdlib.h >
int main() { // pointer to an array
// here arr is the pointer which points to whole array
char( * arr)[] = "hklpo"; // will emit warning. or some compilers may not support.
printf("%c\n", ( * arr)[0]);
// array of pointers
// here str contains the pointers and itself is array
// its index will point to specific element
char * str[] = {
"dafds",
"DFdf"
};
printf("%s\n", str[1]);
// how integers work
// pointer to array of integers
int arrInt[] = {
1,
2,
3,
4,
5
};
int( * stra)[] = arrInt; // cannot directly give values like we did in char array.
// Because strings are stored as array internally. But we can also assign char array as we did in integer array.
printf("%d\n", ( * stra)[2]);
// array of integer pointers
int * arrIntt[] = {
1,
2,
3,
4,
5
};
printf("%d\n", arrIntt[1]);
// size of check
// remember a pointer size if 8 bytes on a 64 bit machine and 4 bytes on 32 bit machine
// because pointer stores the address
printf("\nSize check : \nChar Pointer to Array: %d,\nArray of Char pointer: %d,\n\
Integer Pointer to Array: %d,\nArray of integer Pointers: %d\n\n", sizeof(arr), sizeof(str), sizeof(stra), sizeof(arrIntt));
// see below for pointer size vs data type size
char * a = 'a';
printf("%d", sizeof(a)); // give 8 byte on 64 bit machine
char b = 'b';
printf("%d", sizeof(b)); // give 1 byte on most of machine (language dependent)
return 0;
}
Pointer to Array Vs Array of Pointer in C
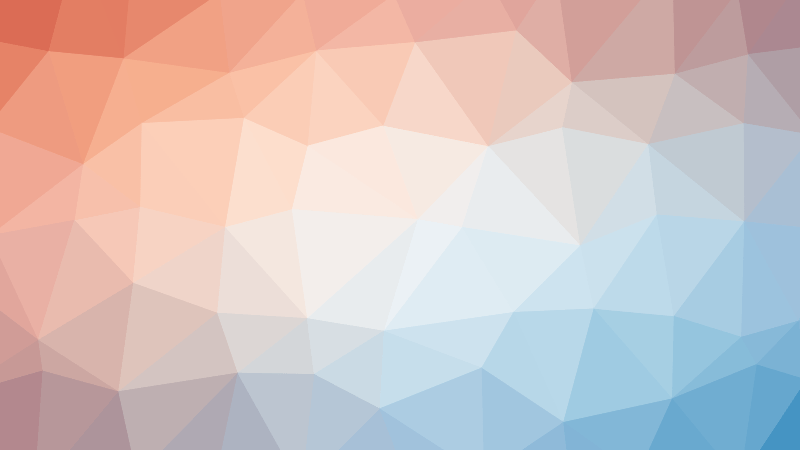