Problem statement
You are given four integers sx
, sy
, fx
, fy
, and a non-negative integer t
.
In an infinite 2D grid, you start at the cell (sx, sy)
. Each second, you must move to any of its adjacent cells.
Return true
if you can reach cell (fx, fy)
after exactly t
seconds, or false
otherwise.
A cell’s adjacent cells are the 8 cells around it that share at least one corner with it. You can visit the same cell several times.
Example 1:
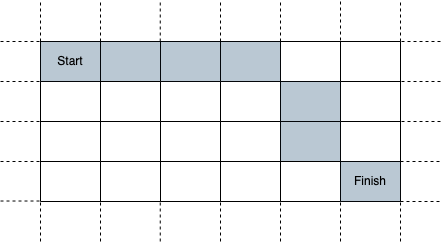
Input: sx = 2, sy = 4, fx = 7, fy = 7, t = 6
Output: true
Explanation: Starting at cell (2, 4), we can reach cell (7, 7) in exactly 6 seconds by going through the cells depicted in the picture above.
Example 2:
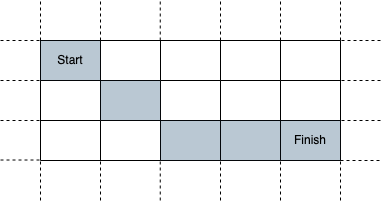
Input: sx = 3, sy = 1, fx = 7, fy = 3, t = 3
Output: false
Explanation: Starting at cell (3, 1), it takes at least 4 seconds to reach cell (7, 3) by going through the cells depicted in the picture above. Hence, we cannot reach cell (7, 3) at the third second.
Constraints:
1 <= sx, sy, fx, fy <= 109
0 <= t <= 109
Solution
public bool IsReachableAtTime(int sx, int sy, int fx, int fy, int t) {
// we need to reach to the nearest point diagonally, then in x or y direction.
// we will try to move maximum diagonally or in x/y direction.
// Special case handling.
// Note: Maybe we can handle it some other way
if (sx == fx && sy == fy && t == 1) {
return false;
}
var maxMovement = 0;
while ((sx != fx || sy != fy) && t > 0) {
// move diagonally to the closet point
if (sx != fx && sy != fy) {
// next sx, sy
if (sx > fx) {
maxMovement = sx - fx;
} else {
maxMovement = fx - sx;
}
if (sy > fy) {
maxMovement = Math.Min(sy - fy, maxMovement);
} else {
maxMovement = Math.Min(fy - sy, maxMovement);
}
// now move maxMovement
if (sx > fx) {
sx -= maxMovement;
} else {
sx += maxMovement;
}
if (sy > fy) {
sy -= maxMovement;
} else {
sy += maxMovement;
}
t-=maxMovement;
} else if (sx == fx) {
// move in y direction
if (sy > fy) {
maxMovement = sy - fy;
t-= maxMovement;
sy-= maxMovement;
} else {
maxMovement = fy - sy;
t-= maxMovement;
sy+= maxMovement;
}
} else if (sy == fy) {
// move in x direction
if (sx > fx) {
maxMovement = sx - fx;
t-= maxMovement;
sx-= maxMovement;
} else {
maxMovement = fx - sx;
t-= maxMovement;
sx+= maxMovement;
}
}
maxMovement = 0;
}
return sx == fx && sy == fy && t >= 0;
}